在 Python 的世界里,`start` 并非一个独立的函数,而是通常与其他函数或方法结合使用,赋予它们独特的行为。想要理解 `start` 的用法,我们必须先了解它在不同场景中的角色。
1. 启动线程: `threading.Thread.start()`
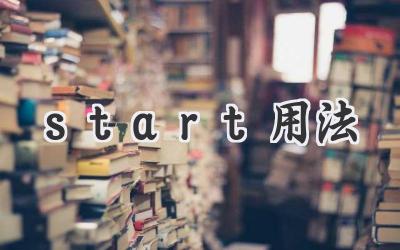
当我们需要同时执行多个任务时,线程便成为了利器。`threading.Thread.start()` 方法正是用来启动一个新的线程的。它会创建一个新的线程,并开始执行该线程中的目标函数。
```python
import threading
def worker_function():
print("Worker thread is running!")
# 创建一个线程
thread = threading.Thread(target=worker_function)
# 启动线程
thread.start()
print("Main thread is running!")
```
在这个例子中,`worker_function` 会在独立的线程中执行,而主线程则继续运行。
2. 启动进程: `multiprocessing.Process.start()`
与线程不同,进程拥有独立的内存空间,可以更好地处理计算密集型任务。`multiprocessing.Process.start()` 方法负责启动一个新的进程,并将目标函数运行在该进程中。
```python
import multiprocessing
def worker_function():
print("Worker process is running!")
# 创建一个进程
process = multiprocessing.Process(target=worker_function)
# 启动进程
process.start()
print("Main process is running!")
```
类似于线程,`worker_function` 会在新的进程中执行,而主进程则继续运行。
3. 启动服务: `socket.socket.listen()`
在网络编程中,`socket.socket.listen()` 函数扮演着启动服务器的重要角色。它会将一个套接字设置为监听状态,等待来自客户端的连接请求。
```python
import socket
# 创建一个套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 绑定地址和端口
sock.bind(('localhost', 8080))
# 设置监听,最大连接数量为 5
sock.listen(5)
# 接收客户端连接
conn, addr = sock.accept()
# 与客户端通信
print(f'Connected by {addr}')
conn.sendall(b'Welcome to the server!')
conn.close()
```
`listen()` 函数会使套接字开始监听,并准备接收来自客户端的连接。
4. 启动定时器: `threading.Timer.start()`
`threading.Timer.start()` 函数可以用来创建一个定时器,在指定的时间间隔后执行目标函数。
```python
import threading
import time
def hello_world():
print("Hello, world!")
# 创建一个定时器,延迟 3 秒执行 hello_world
timer = threading.Timer(3, hello_world)
# 启动定时器
timer.start()
# 主线程继续运行
print("Main thread is running...")
time.sleep(5)
```
在这个例子中,`hello_world` 函数会在 3 秒后执行,而主线程则继续运行。
拓展:start 函数的更多应用
除了上述常见的场景,`start` 也可以在其他库和框架中发挥作用。例如,在 Flask 框架中,`app.run()` 函数用于启动一个 Web 服务器,而 `app.start()` 函数则是用来启动一个 Flask 应用的底层方法。
总而言之,`start` 在 Python 中扮演着启动和运行各种任务的角色,它与其他函数和方法的配合,构成了 Python 强大功能的基础。 了解 `start` 的用法,将帮助你更好地掌握 Python 的编程技巧。
评论